Given an m x n
matrix
, return all elements of the matrix
in spiral order.
Example 1:
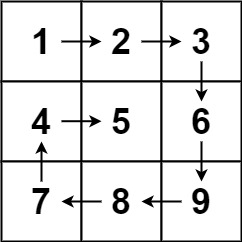
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]]
Output: [1,2,3,6,9,8,7,4,5]
Example 2:
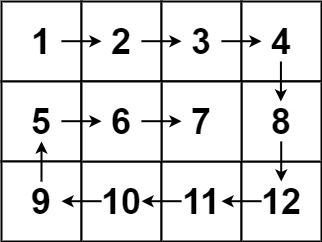
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]]
Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 10
-100 <= matrix[i][j] <= 100
Program
class Solution {
public:
vector<int> spiralOrder(vector<vector<int>>& matrix) {
int left = 0, right = matrix[0].size(); // Columns
int top = 0, bottom = matrix.size(); // Rows
vector<int> res;
while (left < right && top < bottom) {
for (int i = left; i < right; i++) {
// Top row
res.push_back(matrix[top][i]);
}
top++;
for (int i = top; i < bottom; i++) {
// Right Column
res.push_back(matrix[i][right - 1]);
}
right--;
if (left >= right || top >= bottom) {
break;
}
for (int i = right-1; i >= left; i--) {
// Bottom Row
res.push_back(matrix[bottom-1][i]);
}
bottom--;
for (int i = bottom-1; i >= top; i--) {
// Left Column
res.push_back(matrix[i][left]);
}
left++;
}
return res;
}
};
Comments
Post a Comment